전체 소스코드
#include <gst/gst.h>
#ifdef __APPLE__
#include <TargetConditionals.h>
#endif
int
tutorial_main (int argc, char *argv[])
{
GstElement *pipeline;
GstBus *bus;
GstMessage *msg;
/* Initialize GStreamer */
gst_init (&argc, &argv);
/* Build the pipeline */
pipeline =
gst_parse_launch
("playbin uri=https://gstreamer.freedesktop.org/data/media/sintel_trailer-480p.webm",
NULL);
/* Start playing */
gst_element_set_state (pipeline, GST_STATE_PLAYING);
/* Wait until error or EOS */
bus = gst_element_get_bus (pipeline);
msg =
gst_bus_timed_pop_filtered (bus, GST_CLOCK_TIME_NONE,
GST_MESSAGE_ERROR | GST_MESSAGE_EOS);
/* See next tutorial for proper error message handling/parsing */
if (GST_MESSAGE_TYPE (msg) == GST_MESSAGE_ERROR) {
g_error ("An error occurred! Re-run with the GST_DEBUG=*:WARN environment "
"variable set for more details.");
}
/* Free resources */
gst_message_unref (msg);
gst_object_unref (bus);
gst_element_set_state (pipeline, GST_STATE_NULL);
gst_object_unref (pipeline);
return 0;
}
int
main (int argc, char *argv[])
{
#if defined(__APPLE__) && TARGET_OS_MAC && !TARGET_OS_IPHONE
return gst_macos_main ((GstMainFunc) tutorial_main, argc, argv, NULL);
#else
return tutorial_main (argc, argv);
#endif
}
코드 설명
// 초기화
gst_init(&argc, &argv);
gst_init()
- 모든 내부 구조 초기화
- 플러그인 확인
- 명령줄 옵션 실행
// 파이프라인 빌드
pipeline =
gst_parse_launch
("playbin uri=https://gstreamer.freedesktop.org/data/media/sintel_trailer-480p.webm", NULL);
Gstreamer
- 멀티미디어 흐름을 처리하도록 설계된 프레임워크
- 미디어는 모든 종류의 작업을 수행하는 일련의 중간 요소를 통과한면서 "소스"(생산자)에서 "싱크"(소비자)까지 이동한다.
- 상호 연결된 모든 요소의 집합을 "파이프라인"이라고 한다.
gst_parse_launch
- 파이프라인이 충분히 쉽고 고급기능이 필요하지 않은 경우 사용
- 파이프라인의 텍스트 표현을 가져와서 실제 파이프라인으로 변환.
playbin(플레이빈)
- 파이프라인 구축 요소
- 소스와 싱크 역할을 하는 특수 요소이자 전체 파이프라인
- 내부적으로 미디어 재생에 필요한 모든 요소를 생성하고 연결해줌.
// 플레이 시작
gst_element_set_state (pipeline, GST_STATE_PLAYING);
상태
- 파이프라인의 상태를 Play 상태로 변경시켜 미디어를 재생.
// 오류가 발생하거나 EOS(End Os Stream)을 찾을 때까지 대기
bus = gst_element_get_bus (pipeline);
msg =
gst_bus_timed_pop_filtered (bus, GST_CLOCK_TIME_NONE,
GST_MESSAGE_ERROR | GST_MESSAGE_EOS);
gst_element_get_bus
- 파이프라인의 bus를 가져옴.
gst_bus_timed_pop_filterd
- bus를 통해 ERROR 또는 EOS(End-of-Stream)을 수신할 때까지 차단됨.
Gstreamer
- 이 시점부터 Gstreamer가 모든 것을 처리
- 미디어가 EOS에 도달하거나 오류(ERROR)가 발생하면 실행이 종료된다.
- 또는 Ctrl-C를 누르면 언제든지 애플리케이션을 중지할 수 있다.
// 리소스 정리(해제)
gst_message_unref (msg);
gst_object_unref (bus);
gst_element_set_state (pipeline, GST_STATE_NULL);
gst_object_unref (pipeline);
return 0;
gst_message_unref (messge)
- gst_bus_timed_pop_filtered에서 사용된 메세지 반환
gst_object_unref (bus)
- gst_element_get_bus로 추가한 버스를 해제
gst_element_set_state (pipeline, GST_STATE_NULL)
- 상태를 NULL 상태로 변경하여 할당한 모든 리소스 해제
gst_object_unref (pipline)
- 파이프라인의 참조 취소
결과
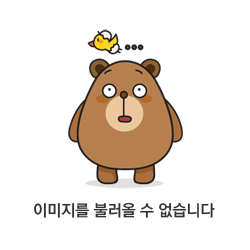
정리
gst_init() : Gstreamer 초기화
gst_parse_launch : 텍스트 설명에서 파이프라인을 빠르게 구축
playbin : 자동 재생 파이프라인을 생성
gst_element_set_state : 재생을 시작하도록 Gstreamer에 신호를 전달
참고자료
Basic tutorial 1: Hello world!
Please port this tutorial to javascript! Basic tutorial 1: Hello world! Goal Nothing better to get a first impression about a software library than to print “Hello World” on the screen! But since we are dealing with multimedia frameworks, we are going
gstreamer.freedesktop.org